HashingΒΆ
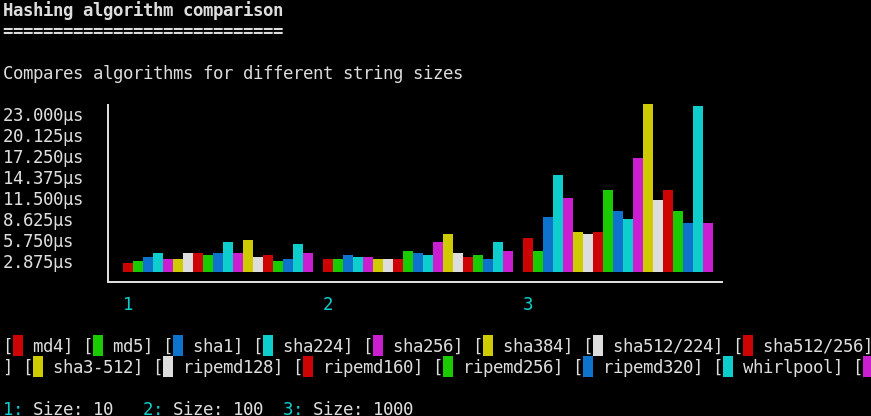
Console Output
This hashing benchmark accepts two parameter sources: one for algorithms and the other for the size of the string to hash.
Parameters from different providers are combined into a cartesian product, meaning that each algorithm will be combined with each size.
/**
* @BeforeMethods("setUp")
*/
class HashingBench
{
/**
* @var string
*/
private $string = '';
public function setUp(array $params): void
{
$this->string = str_repeat('X', $params['size']);
}
/**
* @ParamProviders({
* "provideAlgos",
* "provideStringSize"
* })
*/
public function benchAlgos($params): void
{
hash($params['algo'], $this->string);
}
public function provideAlgos()
{
foreach (array_slice(\hash_algos(), 0, 20) as $algo) {
if ($algo === 'md2') { // md2 is in a different performance category
continue;
}
yield ['algo' => $algo];
}
}
public function provideStringSize() {
yield ['size' => 10];
yield ['size' => 100];
yield ['size' => 1000];
}
}
You can configure a bar chart report grouping both by size and algorithm:
{
"report.generators": {
"hashing": {
"title": "Hashing algorithm comparison",
"description": "Compares algorithms for different string sizes",
"generator": "component",
"filter": "benchmark_name = 'HashingBench'",
"components": [
{
"component": "bar_chart_aggregate",
"x_partition": "'Size: ' ~ variant_params['size']",
"bar_partition": "variant_params['algo']",
"y_expr": "mode(partition['result_time_avg']) as time",
"y_axes_label": "yValue as time"
}
]
}
}
}